Meta Tags and SEO
Nuxt gives you 3 different ways to add meta data to your application:
- Globally using the nuxt.config.js
- Locally using the head as an object
- Locally using the head as a function so that you have access to data and computed properties.
Global Settings
Nuxt lets you define all default <meta>
tags for your application inside the nuxt.config.js file using the head property. This is very useful for adding a default title and description tag for SEO purposes or for setting the viewport or adding the favicon.
export default {
head: {
title: 'my website title',
meta: [
{ charset: 'utf-8' },
{ name: 'viewport', content: 'width=device-width, initial-scale=1' },
{
hid: 'description',
name: 'description',
content: 'my website description'
}
],
link: [{ rel: 'icon', type: 'image/x-icon', href: '/favicon.ico' }]
}
}
Local Settings
You can also add titles and meta for each page by setting the head
property inside your script tag on every page:
<script>
export default {
head: {
title: 'Home page',
meta: [
{
hid: 'description',
name: 'description',
content: 'Home page description'
}
],
}
}
</script>
head
as an object to set a title and description only for the home page<template>
<h1>{{ title }}</h1>
</template>
<script>
export default {
data() {
return {
title: 'Home page'
}
},
head() {
return {
title: this.title,
meta: [
{
hid: 'description',
name: 'description',
content: 'Home page description'
}
]
}
}
}
</script>
head
as a function to set a title and description only for the home page. By using a function you have access to data and computed propertiesNuxt uses vue-meta to update the document head and meta attributes of your application.
hid
key to the meta description. This way vue-meta
will know that it has to overwrite the default tag.head
, in the vue-meta documentation .External Resources
You can include external resources such as scripts and fonts by adding them globally to the nuxt.config.js
or locally in the head
object or function.
body: true
to include the resource before the closing </body>
tag.Global Settings
export default {
head: {
script: [
{
src: 'https://cdnjs.cloudflare.com/ajax/libs/jquery/3.1.1/jquery.min.js'
}
],
link: [
{
rel: 'stylesheet',
href: 'https://fonts.googleapis.com/css?family=Roboto&display=swap'
}
]
}
}
Local Settings
<template>
<h1>About page with jQuery and Roboto font</h1>
</template>
<script>
export default {
head() {
return {
script: [
{
src:
'https://cdnjs.cloudflare.com/ajax/libs/jquery/3.1.1/jquery.min.js'
}
],
link: [
{
rel: 'stylesheet',
href: 'https://fonts.googleapis.com/css?family=Roboto&display=swap'
}
]
}
}
}
</script>
<style scoped>
h1 {
font-family: Roboto, sans-serif;
}
</style>





























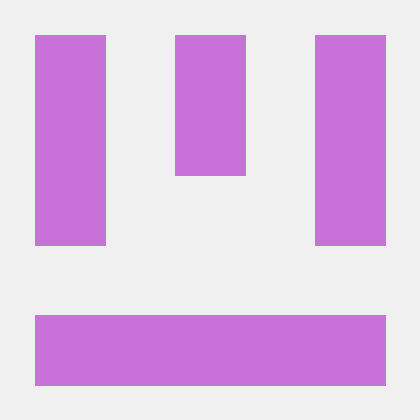














