Enabling Auto-Discovery
Starting from v2.13
, Nuxt can auto import your components when used in your templates. To activate this feature, set components: true
in your configuration:
export default {
components: true
}
Using Components
Once you create your components in the components directory they will then be available throughout your app without the need to import them.
| components/
--| TheHeader.vue
--| TheFooter.vue
<template>
<div>
<TheHeader />
<Nuxt />
<TheFooter />
</div>
</template>
Component Names
If you have components in nested directories such as:
| components/
--| base/
----| foo/
------| Button.vue
The component name will be based on its own path directory and filename. Therefore, the component will be:
<BaseFooButton />
Button.vue
to be BaseFooButton.vue
.)If you want to use a custom directory structure that should not be part of the component name, you can explicitly specify these directories:
| components/
--| base/
----| foo/
------| Button.vue
components: {
dirs: [
'~/components',
'~/components/base'
]
}
And now in your template you can use FooButton
instead of BaseFooButton
.
<FooButton />
Dynamic Imports
To dynamically import a component (also known as lazy-loading a component) all you need to do is add the Lazy
prefix to the component name.
<template>
<div>
<TheHeader />
<Nuxt />
<LazyTheFooter />
</div>
</template>
This is particularly useful if the component is not always needed. By using the Lazy
prefix you can delay loading the component code until the right moment, which can be helpful for optimizing your JavaScript bundle size.
<template>
<div>
<h1>Mountains</h1>
<LazyMountainsList v-if="show" />
<button v-if="!show" @click="show = true">Show List</button>
</div>
</template>
<script>
export default {
data() {
return {
show: false
}
}
}
</script>
Cheatsheet





























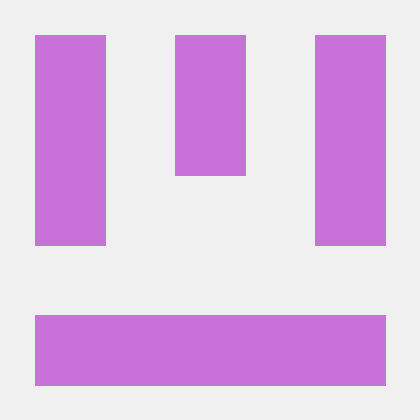














