The server property
Nuxt let you define the server connection variables for your application inside nuxt.config.js
.
-
Type:
Object
Basic example:
export default {
server: {
port: 8000, // default: 3000
host: '0.0.0.0', // default: localhost,
timing: false
}
}
This lets you specify the host and port for your Nuxt server instance.
Example using HTTPS configuration
import path from 'path'
import fs from 'fs'
export default {
server: {
https: {
key: fs.readFileSync(path.resolve(__dirname, 'server.key')),
cert: fs.readFileSync(path.resolve(__dirname, 'server.crt'))
}
}
}
You can find additional information on creating server keys and certificates on localhost
on certificates for localhost article.
Example using sockets configuration
export default {
server: {
socket: '/tmp/nuxt.socket'
}
}
timing
-
Type:
Object
orBoolean
-
Default:
false
Enabling the server.timing
option adds a middleware to measure the time elapsed during server-side rendering and adds it to the headers as 'Server-Timing'
Example using timing configuration
server.timing
can be an object for providing options. Currently, only total
is supported (which directly tracks the whole time spent on server-side rendering)
export default {
server: {
timing: {
total: true
}
}
}
Using timing API
The timing
API is also injected into the response
on server-side when server.time
is enabled.
Syntax
res.timing.start(name, description)
res.timing.end(name)
Example using timing in serverMiddleware
export default function (req, res, next) {
res.timing.start('midd', 'Middleware timing description')
// server side operation..
// ...
res.timing.end('midd')
next()
}
Then server-timing
head will be included in response header like:
Server-Timing: midd;desc="Middleware timing description";dur=2.4
Please refer to Server-Timing MDN for more details.





























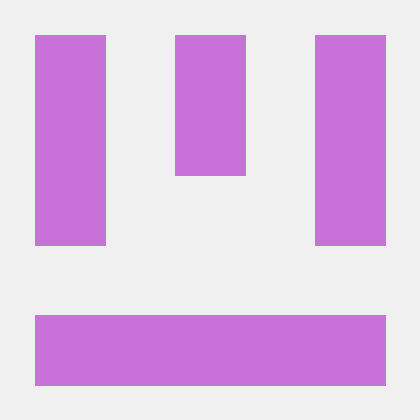














