The render property
Nuxt lets you customize runtime options for rendering pages
bundleRenderer
-
Type:
Object
Use this option to customize vue SSR bundle renderer. This option is skipped if
ssr: false
.
export default {
render: {
bundleRenderer: {
directives: {
custom1(el, dir) {
// something ...
}
}
}
}
}
Learn more about available options on Vue SSR API Reference . It is recommended to not use this option as Nuxt is already providing best SSR defaults and misconfiguration might lead to SSR problems.
etag
-
Type:
Object
-
Default:
{ weak: true }
-
Default:
To disable etag for pages set etag: false
See etag docs for possible options.
You can use your own hash function by specifying etag.hash
:
import { murmurHash128 } from 'murmurhash-native'
export default {
render: {
etag: {
hash: html => murmurHash128(html)
}
}
}
In this case we use murmurhash-native , which is faster for larger HTML body sizes. Note that the weak
option is ignored, when specifying your own hash function.
compressor
-
Type
Object
-
Default:
{ threshold: 0 }
-
Default:
When providing an object, the compression middleware will be used (with respective options).
If you want to use your own compression middleware, you can reference it directly (e.g. otherComp({ myOptions: 'example' })
).
To disable compression, use compressor: false
.
fallback
-
Type
Object
-
Default:
{ dist: {}, static: { skipUnknown: true } }
-
dist
key is for routes matching the publicPath (ie:/_nuxt/*
) -
static
key is for routes matching routes matching/*
-
Default:
dist
andstatic
values are forwarded to serve-placeholder middleware.
If you want to disable one of them or both, you can pass a falsy value.
Example of allowing .js
extension for routing (ex: /repos/nuxt.js
):
export default {
render: {
fallback: {
static: {
// Avoid sending 404 for these extensions
handlers: {
'.js': false
}
}
}
}
}
http2
-
Type
Object
-
Default:
{ push: false, pushAssets: null }
-
Default:
Activate HTTP2 push headers.
You can control what links to push using pushAssets
function.
Example:
pushAssets: (req, res, publicPath, preloadFiles) =>
preloadFiles
.filter(f => f.asType === 'script' && f.file === 'runtime.js')
.map(f => `<${publicPath}${f.file}>; rel=preload; as=${f.asType}`)
You can add your own assets to the array as well. Using req
and res
you can decide what links to push based on the request headers, for example using the cookie with application version.
The assets will be joined together with ,
and passed as a single Link
header.
asyncScripts
-
Type:
Boolean
-
Default:
false
-
Default:
Adds an
async
attribute to<script>
tags for Nuxt bundles, enabling them to be fetched in parallel to parsing (available with2.14.8+
). More information .
injectScripts
-
Type:
Boolean
-
Default:
true
-
Default:
Adds the
<script>
for Nuxt bundles, set it tofalse
to render pure HTML without JS (available with2.8.0+
)
resourceHints
-
Type:
Boolean
-
Default:
true
-
Default:
Adds
prefetch
andpreload
links for faster initial page load time.
You may want to only disable this option if you have many pages and routes.
ssr
-
Type:
Boolean
-
Default:
true
-
false
only client side rendering
-
Default:
Enable SSR rendering
This option is automatically set based on global ssr
value if not provided. This can be useful to dynamically enable/disable SSR on runtime after image builds (with docker for example).
crossorigin
-
Type:
String
-
Default:
undefined
Configure thecrossorigin
attribute on<link rel="stylesheet">
and<script>
tags in generated HTML.
More Info: CORS settings attributes
ssrLog
-
Type:
Boolean
|String
-
Default:
true
in dev mode andfalse
in production
-
Default:
Forward server-side logs to the browser for better debugging (only available in development)
To collapse the logs, use 'collapsed'
value.
static
-
Type:
Object
orfalse
-
Default:
{}
-
Default:
Configure
static/
directory behavior
See serve-static docs for possible options. Alternatively, you can pass false
to disable serve-static
middleware entirely for the static/
directory (for example, if you are hosting these files on a CDN).
Additional to them, we introduced a prefix
option which defaults to true
. It will add the router base to your static assets.
Example:
-
Assets:
favicon.ico
-
Router base:
/t
-
With
prefix: true
(default):/t/favicon.ico
-
With
prefix: false
:/favicon.ico
Caveats:
Some URL rewrites might not respect the prefix.
dist
-
Type:
Object
-
Default:
{ maxAge: '1y', index: false }
-
Default:
Options used for serving distribution files. Only applicable in production.
See serve-static docs for possible options.
csp
-
Type:
Boolean
orObject
-
Default:
false
-
Default:
Use this to configure Content-Security-Policy to load external resources
Prerequisites:
These CSP settings are only effective when using Nuxt with target: 'server'
to serve your SSR application. The Policies defined under csp.policies
are added to the response Content-Security-Policy
HTTP header.
Updating settings:
These settings are read by the Nuxt server directly from nuxt.config.js
. This means changes to these settings take effect when the server is restarted. There is no need to rebuild the application to update the CSP settings.
Debug violations:
If reportOnly
is set to true
(default: false
) the usual header Content-Security-Policy
is set to Content-Security-Policy-Report-Only
. This provides information about violations of the CSP and how to fix them.
HTML meta tag:
In order to add <meta http-equiv="Content-Security-Policy"/>
to the <head>
you need to set csp.addMeta
to true
. Please note that this feature is independent of the csp.policies
configuration:
-
it only adds a
script-src
type policy, and -
the
script-src
policy only contains the hashes of the inline<script>
tags.
When csp.addMeta
is set to true
, the complete set of the defined policies are still added to the HTTP response header.
Note that CSP hashes will not be added as <meta>
if script-src
policy contains 'unsafe-inline'
. This is due to browser ignoring 'unsafe-inline'
if hashes are present. Set option unsafeInlineCompatibility
to true
if you want both hashes and 'unsafe-inline'
for CSPv1 compatibility. In that case the <meta>
tag will still only contain the hashes of the inline <script>
tags, and the policies defined under csp.policies
will be used in the Content-Security-Policy
HTTP response header.
export default {
render: {
csp: true
}
}
// OR
export default {
render: {
csp: {
hashAlgorithm: 'sha256',
policies: {
'script-src': [
'https://www.google-analytics.com',
'https://name.example.com'
],
'report-uri': ['https://report.example.com/report-csp-violations']
},
addMeta: true
}
}
}
// OR
/*
The following example allows Google Analytics, LogRocket.io, and Sentry.io
for logging and analytic tracking.
Review to this blog on Sentry.io
https://blog.sentry.io/2018/09/04/how-sentry-captures-csp-violations
To learn what tracking link you should use.
*/
const PRIMARY_HOSTS = `loc.example-website.com`
export default {
render: {
csp: {
reportOnly: true,
hashAlgorithm: 'sha256',
policies: {
'default-src': ["'self'"],
'img-src': ['https:', '*.google-analytics.com'],
'worker-src': ["'self'", `blob:`, PRIMARY_HOSTS, '*.logrocket.io'],
'style-src': ["'self'", "'unsafe-inline'", PRIMARY_HOSTS],
'script-src': [
"'self'",
"'unsafe-inline'",
PRIMARY_HOSTS,
'sentry.io',
'*.sentry-cdn.com',
'*.google-analytics.com',
'*.logrocket.io'
],
'connect-src': [PRIMARY_HOSTS, 'sentry.io', '*.google-analytics.com'],
'form-action': ["'self'"],
'frame-ancestors': ["'none'"],
'object-src': ["'none'"],
'base-uri': [PRIMARY_HOSTS],
'report-uri': [
`https://sentry.io/api/<project>/security/?sentry_key=<key>`
]
}
}
}
}
shouldPreload & shouldPrefetch
Creates <link> tag with rel="preload"
or rel="prefetch"
for external resources (scripts, styles, etc.).
Default configuration:
export default {
render: {
bundleRenderer: {
shouldPrefetch: () => false,
shouldPreload: (fileWithoutQuery, asType) => ["script", "style"].includes(asType),
},
},
}





























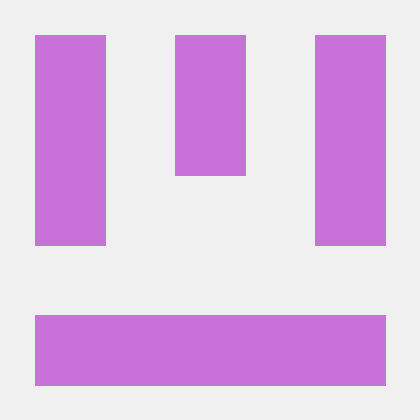














