Middleware directory
The middleware
directory contains your application middleware. Middleware lets you define custom functions that can be run before rendering either a page or a group of pages (layout).
Shared middleware should be placed in the middleware/
directory. The filename will be the name of the middleware (middleware/auth.js
will be the auth
middleware). You can also define page-specific middleware by using a function directly, see anonymous middleware .
A middleware receives the context as the first argument.
export default function (context) {
// Add the userAgent property to the context
context.userAgent = process.server
? context.req.headers['user-agent']
: navigator.userAgent
}
In universal mode, middlewares will be called once on server-side (on the first request to the Nuxt app, e.g. when directly accessing the app or refreshing the page) and on the client-side when navigating to further routes. With ssr: false
, middlewares will be called on the client-side in both situations.
The middleware will be executed in series in this order:
-
nuxt.config.js
(in the order within the file) - Matched layouts
- Matched pages
Router Middleware
A middleware can be asynchronous. To do this return a Promise
or use async/await.
import http from 'http'
export default function ({ route }) {
return http.post('http://my-stats-api.com', {
url: route.fullPath
})
}
Then, in your nuxt.config.js
, use the router.middleware
key.
export default {
router: {
middleware: 'stats'
}
}
Now the stats
middleware will be called for every route change.
You can add your middleware (even multiple) to a specific layout or page as well.
export default {
middleware: ['auth', 'stats']
}
Named middleware
You can create named middleware by creating a file inside the middleware/
directory, the file name will be the middleware name.
export default function ({ store, redirect }) {
// If the user is not authenticated
if (!store.state.authenticated) {
return redirect('/login')
}
}
<template>
<h1>Secret page</h1>
</template>
<script>
export default {
middleware: 'authenticated'
}
</script>
Anonymous middleware
If you need to use a middleware only for a specific page, you can directly use a function for it (or an array of functions):
<template>
<h1>Secret page</h1>
</template>
<script>
export default {
middleware({ store, redirect }) {
// If the user is not authenticated
if (!store.state.authenticated) {
return redirect('/login')
}
}
}
</script>





























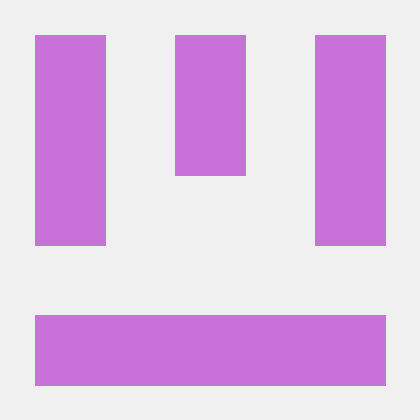














