Layouts directory
Layouts are a great help when you want to change the look and feel of your Nuxt app. Whether you want to include a sidebar or have distinct layouts for mobile and desktop.
Default Layout
You can extend the main layout by adding a layouts/default.vue
file. It will be used for all pages that don't have a layout specified. Make sure to add the <Nuxt>
component when creating a layout to actually include the page component.
All you need in your layout is three lines of code which will render the page component.
<template>
<Nuxt />
</template>
You can add more components here such as Navigation, Header, Footer etc.
<template>
<div>
<TheHeader />
<Nuxt />
<TheFooter />
</div>
</template>
Custom Layout
Every file (top-level) in the layouts
directory will create a custom layout accessible with the layout
property in the page components.
Let's say we want to create a blog layout and save it to layouts/blog.vue
:
<template>
<div>
<div>My blog navigation bar here</div>
<Nuxt />
</div>
</template>
Then you have to tell the pages to use your custom layout
<script>
export default {
layout: 'blog',
// OR
layout (context) {
return 'blog'
}
}
</script>
Error Page
The error page is a page component which is always displayed when an error occurs (that is not thrown on the server-side).
layouts
folder, it should be treated as a page.As mentioned above, this layout is special and you should not include <Nuxt>
inside its template. You must see this layout as a component displayed when an error occurs (404
, 500
, etc.). Similar to other page components, you can set a custom layout for the error page as well in the usual way.
You can customize the error page by adding a layouts/error.vue
file:
<template>
<div class="container">
<h1 v-if="error.statusCode === 404">Page not found</h1>
<h1 v-else>An error occurred</h1>
<NuxtLink to="/">Home page</NuxtLink>
</div>
</template>
<script>
export default {
props: ['error'],
layout: 'blog' // you can set a custom layout for the error page
}
</script>





























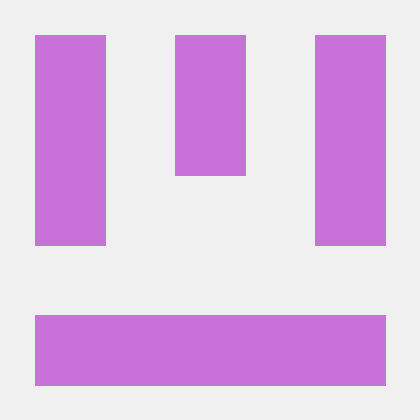














